In this puzzle, we are going to learn how to join dirty data using the SQL Server. There are many instances that you are forced to use someone else’s data. Since it is their system and data (not yours), then you can’t always fix the inconsistently formatted data before it is in the database.
So how can you use it? Do this puzzle and learn how!
Solving puzzles is a great way to learn SQL. Nothing beats practicing what you’ve learned. Once you have figured out the puzzle, post your answer in the comments section so we all can learn from one another.
How Do You JOIN Dirty Data in SQL Server?
In business, the data you get to work with isn’t always clean. For instance, I know of a major automotive manufacturer, whose part number prefix and suffix are separated with dashes in their engineering system, and spaces in their EDI system (e.g. ‘1234-4567’ versus ‘1234 4567’). This makes it difficult to compare and join data from the two system. But in today’s puzzle, we’re going to explore this issue and learn how to do the joins.
For our puzzle we’ll use these tables:
DECLARE @PartDescription TABLE ( PartNumber Varchar(20), Name Varchar(20) ) DECLARE @PartSales TABLE ( PartNumber Varchar(20), Year int, Sales float )
Your goal is to get a result showing the PartNumber, Name, Year, and Sales. Then, sort your results by Name and Year.
Also, the PartNumber you display should have dashes separating the prefix (first four characters) from the suffix (last four characters).
Keep in mind the part number data is inconsistently formatted. You can find the sample data a here.
JOIN Dirty Data – No Results!
Let’s see what happens when we try to join the data without first cleaning it. In a perfect world, we’d expect the correct results however since the part number is in consistently formatted, the join condition fails and the query returns no results.
SELECT P.PartNumber,
P.NAme,
S.Year,
S.Sales
FROM @PartDescription P
INNER JOIN @PartSales S
ON P.PartNumber = S.PartNumber
ORDER BY P.Name, S.Year
Obviously our data needs a good cleaning.
Getting Results with Dirty Data using REPLACE
One way to solve the puzzle is to replace the space and asterisk with a dash. Here is a query to do that:
SELECT REPLACE(REPLACE(P.PartNumber,'*','-'), ' ','-') as PartNumber, P.NAme, S.Year, S.Sales FROM @PartDescription P INNER JOIN @PartSales S ON REPLACE(REPLACE(P.PartNumber,'*','-'), ' ','-') = REPLACE(REPLACE(S.PartNumber,'*','-'), ' ','-') ORDER BY P.Name, S.Year
In this example we used the REPLACE function to search for incorrect characters, such as the asterisk and space, and substituted them with a dash.
You’ll notice that the REPLACE functions are nested. This allows us to get around a limitation with REPLACE.
Here is the result of the join once the data is cleansed.
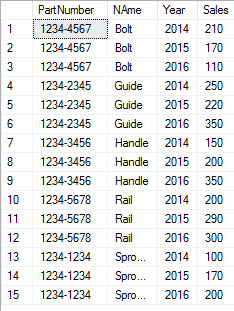
There is a flaw in this method. That is, you need to know which characters you wish to cleanse or clear. In our case, we know that spaces and asterisks are unwanted, but, what if we encounter an equal sign?
The way our solution is written, this would not be found and replaced with a dash. Therefore, the join condition would fail.
In addition, as you add more characters to search, you need to rest more REPLACE functions. It can become quite cumbersome after a while to read and be more error-prone to write.
In a future version of SQL, we’ll be able to use TRANSLATE. Then, I’m hoping we can replace all special characters as so:
SELECT REPLACE(REPLACE(P.PartNumber,'*','-'), ' ','-') as PartNumber, P.NAme, S.Year, S.Sales FROM @PartDescription P INNER JOIN @PartSales S ON TRANSLATE(P.PartNumber,'!@#$%^&*()_+=','-------------') = TRANSLATE(S.PartNumber, '!@#$%^&*()_+=','-------------') ORDER BY P.Name, S.Year
JOIN Dirty Data by Parsing the Part Number
Another way to solve is this problem is by parsing. That is, we can separate the part number into the prefix and suffix. Once done, we join on these to match rows from the two tables.
Here is a diagram of the part number structure. In our example the prefix is the first four characters, and the suffix the last four.
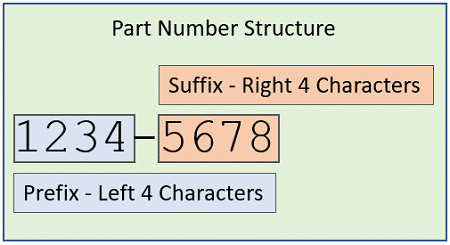
The prefix is calculated using the LEFT function. LEFT(P.PartNumber,4) returns the first four characters. Likewise, RIGHT(P.PartNumber,4) returns the last four characters.
Now, all we need to do is write the join condition to compare the prefix and suffix from each table. Check out this query to see what I mean:
SELECT REPLACE(REPLACE(P.PartNumber,'*','-'), ' ','-') as PartNumber,
P.NAme,
S.Year,
S.Sales
FROM @PartDescription P
INNER JOIN @PartSales S
ON LEFT(P.PartNumber,4) = LEFT(S.PartNumber,4) AND
RIGHT(P.PartNumber,4) = RIGHT(S.PartNumber,4)
ORDER BY P.Name, S.Year
Conclusion
There are certainly other ways to solve this puzzle. I hope that by seeing the two solutions here, you have seen that there are two drastically different approaches to the answer.
If you have a good answer, let me know. I would love to see it!